This HTML cheatsheet covers a variety of tags and attributes, including some newer HTML5 features. Keep in mind that the web development landscape is continually evolving, so staying up-to-date with the latest HTML standards is essential for creating modern and accessible web content.
Syntax
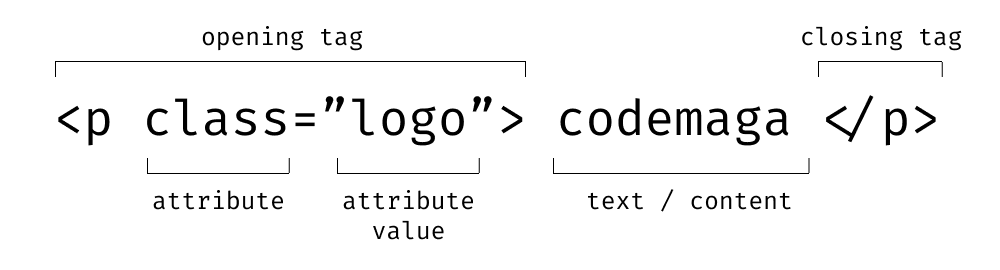
Basic Tags
<!-- Create an HTML document -->
<html> </html>
<!-- Includes all the meta data that isn't displayed -->
<head> </head>
<!-- The visible portion of the document -->
<body> </body>
<!-- Name of the document -->
<title> </title>
<!--
Comments can be
multiple lines long.
-->
Document Structure / Boilerplate
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>Document</title>
</head>
<body>
<!-- Content goes here -->
</body>
</html>
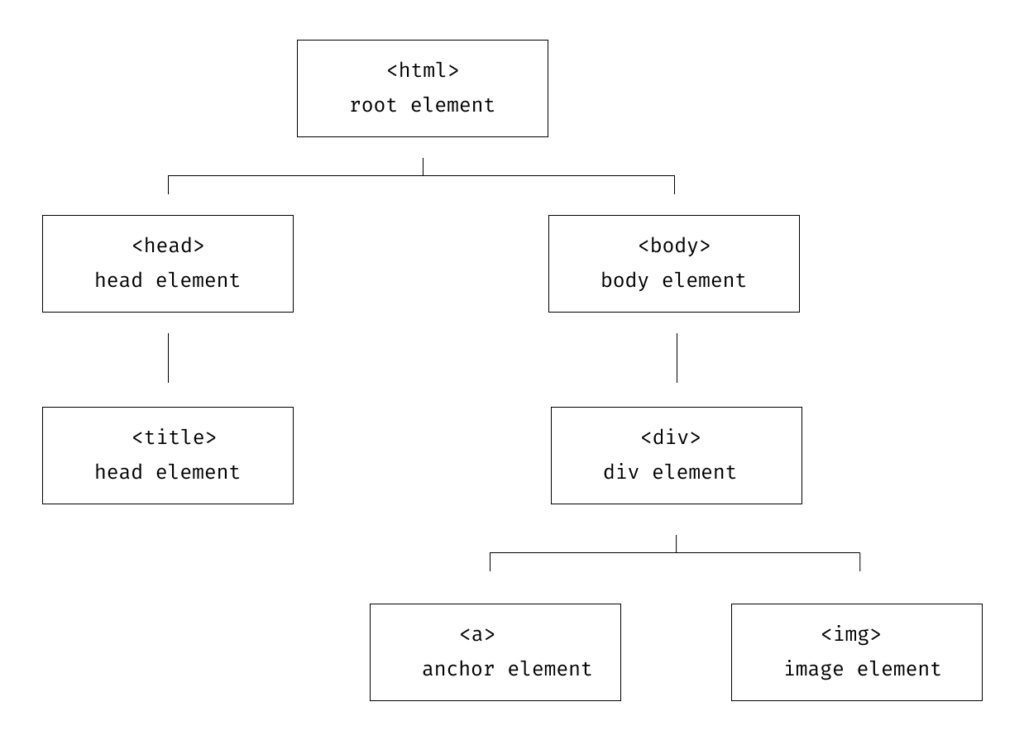
Formatting: Headings, Paragraphs
<!-- Headings (h1 to h6) -->
<h1>Heading 1</h1>
<h2>Heading 2</h2>
...
<h6>Heading 6</h6>
<!-- Paragraph -->
<p>Text in a paragraph.</p>
<!-- Bold and Italic -->
<strong>Bold Text</strong>
<em>Italic Text</em>
<!-- Puts content in a quote indents -->
<blockquote cite="https://en.wikipedia.org/wiki/HTML">The HyperText Markup Language or HTML is the standard markup language for documents designed to be displayed in a web browser.</blockquote>
Lists
<!-- Unordered List -->
<ul>
<li>Item 1</li> <!-- list item -->
<li>Item 2</li>
</ul>
<!-- Ordered List -->
<ol start="3">
<li>Item 3</li>
<li>Item 4</li>
</ol>
<!-- Definition List -->
<dl>
<dt>Title 1</dt>
<dd>Description 1</dd>
</dl>
Links and Images
<!-- Anchor (Link) -->
<a href="https://www.example.com" target="_blank" title="Visit Example.com">Visit Example.com</a>
<a href="mailto:EMAIL_ADDRESS">email address</a>
<!-- Image -->
<img src="image.jpg" alt="Description" width="300" height="200">
<!-- Creating image links -->
<a href="https://www.example.com">
<img src="logo.jpg">Click this image
</a>
<!-- Responsive Image -->
<picture>
<source srcset="image.webp" type="image/webp">
<img src="image.jpg" alt="Description" style="width:auto;">
</picture>
<!-- Figure and Figcaption -->
<figure>
<img src="image.jpg" alt="Description">
<figcaption>Caption for the image.</figcaption>
</figure>
<!-- Embed External Content -->
<iframe src="https://www.youtube.com/embed/video-id" width="560" height="315" frameborder="0" allowfullscreen></iframe>
Audio and Video
<!-- Audio Player -->
<audio controls>
<source src="audio.mp3" type="audio/mp3">
Your browser does not support the audio tag.
</audio>
<!-- Video Player -->
<video width="320" height="240" controls>
<source src="movie.mp4" type="video/mp4">
<track default kind="captions" srclang="en" src="movie.srt"/>
Your browser does not support the video tag.
</video>
<!--Embed tag-->
<embed src="babyyoda.gif"/>
Tables
<!-- Table -->
<table border=? cellspacing=? cellpadding=?>
<caption>Details about Tables in HTML</caption>
<thead>
<tr>
<th scope="col">Header 1</th>
<th scope="col" colspan=2>Header 2</th>
</tr>
</thead>
<tbody>
<tr>
<td rowspan=?>Row 1, Cell 1</td>
<td nowrap>Row 1, Cell 2</td>
</tr>
</tbody>
</table>
Layouts
<!-- format block content with id attribute -->
<div id="main-section"></div>
<!-- Heading with id attribute -->
<span>Span</span>
<!-- Line Break -->
<br>
<!-- Horizontal Line -->
<hr size=? width=? noshade>
CSS Styling
<!-- link tag goes inside head tag -->
<link rel="stylesheet" type="text/css" href="styles.css">
<!-- Styles (CSS) -->
<style>
/* CSS styles go here */
body {
font-family: 'Arial', sans-serif;
color: #333;
}
</style>
Forms
<!-- A Typical Form -->
<form action="/submit" method="post" autocomplete="on">
<legend>Login form:</legend>
<!-- Input Field -->
<input type="text" name="username" placeholder="Username" required maxlength="20" pattern="[A-Za-z]+">
<!-- Password Field -->
<input type="password" name="password" placeholder="Password" minlength="8">
<!-- Grouped submit and rest Buttons -->
<fieldset>
<button type="submit">Submit</button>
<button type="reset" disabled></button>
</fieldset>
</form>
<!-- Upload Form with Attributes -->
<form action="/submit" method="post" enctype="multipart/form-data">
<label for="file-upload"></label
<!-- File Input -->
<input id="file-upload" type="file" accept=".jpg, .png" />
</form>
<!-- Output -->
<form oninput="result.value=parseInt(a.value)+parseInt(b.value)">
<input type="range" id="a" value="50"> +
<input type="number" id="b" value="50">
= <output name="result" for="a b">100</output>
</form>
Form Elements
<!-- Textarea -->
<textarea rows="4" cols="50" placeholder="Enter your message..."></textarea>
<!-- Radio Buttons -->
<input type="radio" id="radio1" name="gender" value="male" checked><!-- pre-checked -->
<label for="radio1">Male</label>
<input type="radio" id="radio2" name="gender" value="female">
<label for="radio2">Female</label>
<!-- Checkboxes -->
<input type="checkbox" id="checkbox1" name="interest" value="music" checked> <!-- pre-checked -->
<label for="checkbox1">Music</label>
<input type="checkbox" id="checkbox2" name="interest" value="sports">
<label for="checkbox2">Sports</label>
<!-- Range Input -->
<input type="range" name="volume" min="0" max="100">
<!-- Search Input -->
<input type="search" name="query" placeholder="Search">
<!-- URL Input -->
<input type="url" name="website" placeholder="Enter URL">
<!-- Date Input -->
<input type="date" name="birthdate">
<!-- Select Dropdown -->
<select id="browsers">
<optgroup label="Apple only">
<option value="safari">Safari</option>
</optgroup>
<optgroup label="All">
<option value="chrome">Chrome</option>
<option value="firefox">Firefox</option>
</optgroup>
</select>
<!-- Datalist -->
<datalist>
<option value="Chrome">
<option value="Safari">
<option value="Firefox">
</datalist>
Meter, Progress, Time and Date
<!-- Meter -->
<meter value="70" min="0" max="100">70%</meter>
<!-- Progress -->
<progress value="50" max="100"></progress>
<!-- Time and Date -->
<time datetime="2023-01-01">January 1, 2023</time>
Semantic HTML with structure
<!-- Header Area -->
<header>
<h1>Website Title</h1>
<!-- Navigation area -->
<nav>
<a href="/html/">Learn HTML</a> |
<a href="/css/">Learn CSS</a>
</nav>
</header>
<!-- Primary Content area -->
<main role="main">
<div>
<!-- Article -->
<article>
<h2>Title 1</h2>
<p>Article content goes here.</p>
</article>
<article>
<h2>Title 2</h2>
<p>Article content goes here.</p>
</article>
</div>
<!-- Related section area -->
<section>
<h2>Heading 1</h2>
<h2>Heading 2</h2>
<h2>Heading 3</h2>
</section>
</main>
<!-- Sidebar Area -->
<aside>
<h2>Newletter</h2>
</aside>
<!-- Footer Area -->
<footer>
<p>© 2023 codemaga.com</p>
<address>Contact <a href="mailto:[email protected]">me</a></address>
</footer>
Common Meta Tags
<!-- Charset -->
<meta charset="UTF-8">
<!-- Viewport for Responsive Design -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Description Meta Tag -->
<meta name="description" content="A concise and informative description of the page">
<!-- Keywords Meta Tag (Deprecated) -->
<meta name="keywords" content="HTML, CSS, JavaScript">
<!-- Author Meta Tag -->
<meta name="author" content="Your Name">
JavaScript Integration
<!-- add JavaScript using script tag -->
<script src="script.js" defer></script>
<script>
alert('Hello, World!');
</script>
<!-- inline -->
Canvas for Graphics
<!-- Canvas for Graphics -->
<canvas id="myCanvas" width="200" height="100" style="border:1px solid #000;"></canvas>
<script>
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.fillStyle = "#FF0000";
ctx.fillRect(0, 0, 150, 75);
</script>
Additional Formatting elements
<!-- Paragraph with Highlighted Text using mark tag -->
<p>This is <mark>highlighted</mark> text in a paragraph.</p>
<!-- Representing x squared -->
<p>x<sup>2</sup></p>
<!-- Representing 2 hydrogen atoms bonded to 1 oxygen atom -->
<p>H<sub>2</sub>O</p>
<!-- Paragraph with Strikethrough Text -->
<p><s>Strikethrough Text</s></p>
<!-- Inline quotation -->
<q>How you doin'?</q> from Friends
<p><del>Old</del> <ins>New</ins> Example</p>
<p>Used to represent the <small>small print </small>of a document.</p>
<!-- Creates preformatted text -->
<pre>
This message which is contained in a HTML pre tag
will be shown in a fixed size
font. Also,spaces and
line breaks are preserved as it is.
</pre>
<!-- Code format -->
<code> </code>
<!-- Abbreviation -->
<p><abbr title="Hypertext Markup Language">HTML</abbr> is fun!</p>
<!-- Textual citation -->
<p><cite>"May the Force be with you"</cite> from Star Wars (1977)</p>
<!-- Provides contact information for a person, people, or an organization. -->
<address>
Written by <a href="mailto:[email protected]">peignit</a>.<br>
Find us at:<br>
codemaga.com<br>
Bangalore<br>
India
</address>
<area>
Global Attributes
<!-- Global Attributes -->
<div id="uniqueId" class="highlight" title="Tooltip" style="color: blue;" dir="ltr" lang="en" tabindex="0" contenteditable="true" spellcheck="true" data-CUSTOM-KEY="VALUE" data-category-id="123"></div>
Details and Summary (Toggle Content)
<!-- Details and Summary -->
<details>
<summary>Click to expand</summary>
<p>Hidden content goes here</p>
</details>
Details and Dialog (Modal)
<!-- Dialog -->
<dialog open>
<p>This is a modal dialog.</p>
<button onclick="this.parentElement.close()">Close</button>
</dialog>
Web Components
<!-- Web Components -->
<my-custom-element></my-custom-element>
<!-- Shadow DOM -->
<style>
my-custom-element {
display: block;
border: 1px solid #000;
}
</style>
Best Practices
- Properly indenting your code makes it more readable.
- Make sure to close all of your HTML tags.
Responsive Design
<!-- Viewport Meta Tag for Responsive Design -->
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<!-- Media Queries for Responsive Styles -->
<style>
@media screen and (max-width: 600px) {
body {
background-color: lightblue;
}
}
</style>
Accessibility
Make your webpage accessible to all users with these best practices.
<img src="image.jpg" alt="Description of Image">
<label for="name">Name:</label> <input type="text" id="name" name="name">
Open Graph tags for SEO
<meta property="og:title" content="HTML Cheat Sheet" />
<meta property="og:type" content="website" />
<meta property="og:url" content="https://codemaga.com/" />
<meta property="og:image" content="https://codemaga.com/image.png" />
<!-- optional -->
<meta property="og:site_name" content="HTML CheatSheet" />
<meta property="og:description" content="A brief description" />
<meta property="og:determiner" content="the" />
<meta property="og:locale" content="en_US" />
<meta property="og:locale:alternate" content="es_HI" />
<meta property="og:audio" content="https://codemaga.com/track.mp3" />
<meta property="og:video" content="https://codemaga.com/video.mp4" />
Characters and Symbols
Certain characters in HTML have special meanings and are reserved. Here are some commonly used ones:
Symbol | Description | Entity Name | Number Code |
---|---|---|---|
© | Copyright | © | © |
& | Ampersand | & | & |
> | Greater than | > | > |
< | Less than | < | < |
$ | Dollar | $ | $ |
“ | Quotation mark | " | " |
‘ | Apostrophe | ' | ' |
Note: Not all attributes are applicable to every tag, so it’s important to refer to documentation and specifications for more details.